LJC Community Talk: "How Should Java Developers Build Front-Ends for Web, Mobile & Desktop Today?"
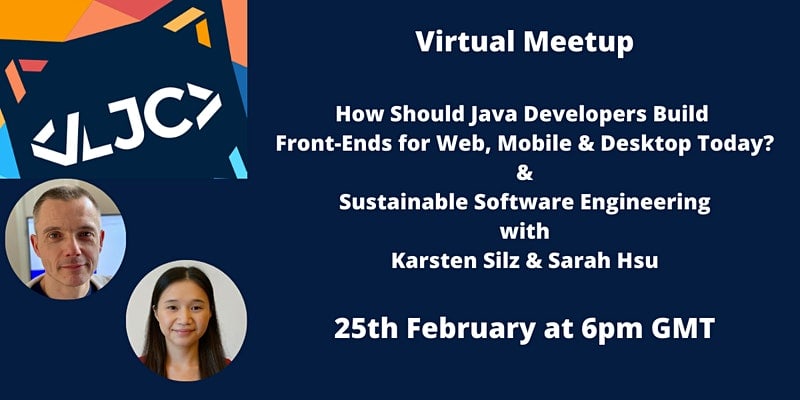
Table Of Contents
- Table Of Contents
- Talk
- Abstract
- Why Should You Listen To Me?
- Slides
- Videos
- Looking For Project in October 2022!
- Additional Talk Information
- Getting Started
- Making Of
Talk
The London Java Community (LJC) regularly hosts community talks. There, two speakers each have 30 minutes for their talks. On Thursday, February 25, 2021, I will present “How Should Java Developers Build Front-Ends for Web, Mobile & Desktop Today?”. I will look at various frameworks from the perspective of a Java developer and suggest which toolkits to use in typical scenarios. Please see the abstract below.
I want to thank Dominique Carlo and Barry Cranford from RecWorks. They make these community talks possible! So if you need to hire Java developers in the U.K., please consider RecWorks!
Abstract
Users access applications on PCs and mobile devices today. There are two obvious ways to build front-ends for these devices: Web applications and native applications. Cross-platform UI toolkits combine advantages from both approaches. Examples are Facebook’s React Native, Google’s Flutter, Microsoft’s Xamarin, and JavaFX. Important web application frameworks are Facebook’s React, Google’s Angular, and Vue.js. I will look at all these frameworks from the perspective of a Java developer and suggest which one to use in three common scenarios.
In 2019, I developed a mobile app prototype with Flutter and a progressive web application prototype. The videos are below. I then decided to use Flutter for native mobile apps in my current project. Based on my experiences, I will highlight typical Flutter issues and how to solve them.
Why Should You Listen To Me?
I’ve been a Java developer for 22 years. As a full-stack developer, I’m about to put my third project into production since 2017. I was the sole developer on two of them and the lead developer on the third one.
I’m not affiliated with the projects I’m discussing here. I’m not selling books or training courses. I’m just sharing industry analysis and my project experiences to give you options for your next project. You then need to apply your own criteria, evaluate these options in your own environment, and make your own choices.
Slides
Here are the slides as PDF. They are 3 MB:
You can also get the slides in their original Keynote format. “Keynote” is Apple’s presentation application. Why would you do that? I animated the slides to better convey information and to make them more pleasant to watch. Or maybe you want to peek under the hood to see how I achieved specific effects. These slides include a video and are 14 MB in size!
Videos
Talk
The talk is on YouTube. It’s 41 minutes long, followed by nearly ten minutes of questions.
Mobile App Prototype with Flutter
In the summer of 2019, I built native iOS/Android apps with Flutter to validate a business problem. It took me about six weeks, and it was my first Flutter project. I used Google’s cloud service Firebase for login, No-SQL database, and file storage. I also built my own back-end with Java, JHipster, Spring Boot, and Angular. This video has the details.
Progressive Web Application Prototype
At the end of 2019, I built a progressive web app (PWA) to speed up app development. A PWA uses the “Service Worker” in a browser to install on your device and cache data. That was about four weeks, and it was my first PWA. I used Google Workbox for this, but developed my own offline storage solution in the browser. I built my back-end with Java, JHipster, Spring Boot, and Angular. This video has the details.
Developer job ads down 32% year over year, Stack Overflow questions dropped 55% since ChatGPT. I now recommend IntelliJ Community Edition because many AI code assistants don't run in Eclipse. Job ads for Quarkus hit an all-time high.
See All Issues & Subscribe
Looking For Project in October 2022!
And now for some shameless self-promotion: I’m looking to join a project in October 2022, in Milton Keynes, London, or remote. I’ll work as a contractor or fixed-term employee, but don’t take permanent positions. Interested? Then check out my resume & work samples!
Additional Talk Information
Declarative Front-Ends
Apple’s SwiftUI
SwiftUI is Apple’s take on declarative front-ends. Here’s the counter example from the talk, with slightly changed formatting:
Google’s Flutter
Flutter is Google’s cross-platform implementation of declarative front-ends. It reached the stable version 1.0 for mobile in December 2018. Here’s what the SwiftUI counter sample looks like in Flutter:
Google’s Jetpack Compose
Jetpack Compose is Google’s Android implementation of declarative front-ends. So Google has two different horses in this race: Jetpack Compose and Flutter. Of course, it’s Google! 😒
Jetpack Compose entered beta on February 24, 20201. According to Google, it now has stable APIs and is feature-complete.
I adopted the counter sample in this tutorial to look like the SwiftUI sample above:
Microsoft’s .NET Multi-platform App UI (MAUI)
.NET MAUI is part of .NET 6, expected for November 2021. And if “Maui” rings a bell for you - it’s the second-largest island of Hawaii.
Microsoft calls its implementation of declarative front-ends “Model-View-Update” (MVU). Here’s what I think the SwiftUI sample from above will look like in MVU. I adapted the sample from the announcement post:
Facebook’s React
And finally, here’s what the counter looks like in Facebook’s React for web applications. I adapted it from this Stackblitz sample. You see some HTML code in there because I don’t use components to keep things simple. If I did, it would look as declarative as the other examples:
Notable Flutter Plugins
- flutter_bootstrap: Make your app responsive with the Bootstrap grid.
- flutter_platform_widgets: Provides UI element abstractions that automatically create either an iOS or Android UI element, depending on the current mobile operating system.
- google_fonts: Adds Google Fonts to your Flutter application.
- url_launcher: Lauch apps to open links, send emails, start phone calls or text messages. You could probably also launch application-specific URLs.
- flutter_email_sender: Send email in-app, using standard iOS/Android components.
- google_maps_flutter: Open Google Maps inside your app. Using Google Maps in your app is free on iOS and Android.
- connectivity: Find out when the network connectivity of your app changes.
- image_picker: Access photos & videos on iOS and Android.
- camera: Access the camera on iOS and Android.
- image_cropper: Edit pictures.
- photo_view: Show pictures with pinch-to-zoom.
- flutter_markdown: Show Markdown.
- native_pdf_view: Show PDF documents.
- flutter_html: Show HTML.
- freezed: Generate immutable classes, complete with JSON serialization.
- flutter_easyloading: Toast overlays for progress & success/info messages.
- package_info: Get the application version number for your “About” screen.
- device_info: Get device information for your “About” screen.
Getting Started
React & JavaScript
The React website is a good starting point. React uses JavaScript to create web applications.
Learning JavaScript
- The MDN Web Docs (the site formerly known as “Mozilla Developer Network”) has various tutorials based on your skill level.
- academind has a JavaScript course with 40 hours of video. The same course is on Udemy, where it may be cheaper in frequent sales.
Learning TypeScript
TypeScript mixes “some Java into JavaScript”, such as types. Hence the name! You have to use TypeScript if you use Angular. And you can use it with React.
You can take a peek in the “TypeScript for Java/C# Programmers” article. If you like it, then the TypeScript handbook is your friend, also in Epub and PDF.
Learning React
- The React site has a tutorial for beginners where you build an app.
- The React site also gives us a tutorial for the React concepts.
- academind has a React course with 40 hours of video, which includes React Hooks and Redux. I’m taking this course right now (March 2021). The same course is on Udemy, where it may be cheaper in frequent sales.
Flutter & Dart
The Flutter website is an excellent place to get familiar with Flutter. Flutter uses the Dart programming language to create natively-compiled applications for mobile, web & desktop. Both Flutter and Dart can use plugins that have a great portal.
Learning Dart
You start with the Dart language tour. Java developers take the “Intro to Dart for Java Developers” next. Then you have options:
- Google has several free tutorials for Dart.
- Code with Andreas has a 10-hour Dart course.
Installing Flutter
Here are the instructions, straight from the Flutter website:
Learning Flutter
Here’s a selection of Flutter tutorials and courses:
- Google has several free tutorials for Flutter.
- freeCodeCamp has at least two free Flutter courses:
- a one-hour course for mobile apps, and
- a three-hour course that claims to include desktop & web, too.
- Code with Andreas has two paid courses:
- a 2.5-hours “Flutter REST API Crash Course” (I took it & liked it a lot!), and
- a 21-hour course on Flutter & Google Firebase.
- Finally, academind has a Flutter course with 41 hours of video. I used the 2019 version to learn Flutter and can highly recommend it! The same course is on Udemy, where it may be cheaper in frequent sales.
Making Of
Third Time’s the Charm?
This is the third time that I gave this talk: The first time was at the LJC Unconference 2020, the second time at the Cincinnati JUG. So I was familiar with it and went from about 50 minutes at the Cincinnati JUG to 41 minutes this time around.
But that’s not enough: For my JavaLand talk, I need to get this down to 35 minutes - in German. So I need to cut nearly 10 minutes because I also want to add some things. 😓
The Summary Stash
I used the “Summary Stash” for the first time ever in a talk. And probably for the last time! But what is that? And why won’t I do this again?
The “Summary Stash” is a slide where I collect miniature versions of important slides for my wrap-up. Since I use Apple Keynote’s Magic Move, it really is a miniature version, not a picture. Here’s an example:
Then at the end, I went through the stash, one slide at a time:
Well, it certainly looks the part. So why won’t I use it again? Three reasons:
- It takes a lot of time in the talk. Between filling the “Summary Stash” and going through it at the end, it takes four minutes in total. Remember, I need to cut nearly ten minutes out!
- I show & talk at the same time. Although my audience has seen the slides before, I still need to give them time to read the slide again. In this talk, I didn’t, violating the “Don’t show & tell at the same time” rule of talks.
- It’s a lot of work to keep the different versions of the “Summary Stash” slide up-to-date. I did change some slides which I then needed to fix on dozens of “Summary Stash” instances.
I have an idea how I could replace the “Summary Stash”, but I’m not sure if will do this.
Out Of Sync
When you give a talk, you have three options to sync-up what your audience sees and hears:
- Show first, then tell: You show your point first on the slide, then talk about it.
- Show & tell: You show your point on the slide and talk about it at the same time. You see this most often, but you shouldn’t do this: People can’t listen and read at the same time, so they either don’t listen to you while reading - or don’t read what you’re showing.
- Tell first, then show: You talk about it your point first and then show it on the slide. That’s what I did here most of the time.
Here’s an example - I talk about a point before it appears on the slide:
This is the second time I did it. And the last time. Why?
- As my wife pointed out when I told her about my talk, it’s confusing to the user. They see a point on the slide while I’m already talking about the next one. Think about subtitles in movies: You see the subtitles first, then you hear the dialog. Listening to your wife is always a good idea. Here it was an especially good one. I only wish I had asked my wife earlier!
- It’s harder to talk about an upcoming point with in your presentation program. Instead of looking at the same screen/content that your audience sees, you need to look at the next screen - on a second screen.
So I’ll go back to option #1 - show first, then tell.
Eyes Up Here!
I always say that you should look directly into the camera in an online talk. Well, where did I look? Not into the camera! Why?
I don’t use the web cam of my Mac. Instead, I use my iPhone with the Reincubate Camo software. And I clearly put it into the wrong place this time. It also didn’t help that the “next slide” that I was talking about was on the right side of my screen (from the audience’s perspective).
I definitely need to record myself before my next talk!
Part 9 of 15
in the Talks
series.
« VJUG Talk: "How Should Java Developers Build Front-Ends for Web, Mobile & Desktop Today?"
|
CinJUG: "How Should Java Developers Build Front-Ends for Web, Mobile, and Desktop Today?" »
| Start: LJC Lightning Talk: Eclipse OpenJ9: Memory Diet for Your JVM Applications
Developer job ads down 32% year over year, Stack Overflow questions dropped 55% since ChatGPT. I now recommend IntelliJ Community Edition because many AI code assistants don't run in Eclipse. Job ads for Quarkus hit an all-time high.
Read my newsletter